The prototypal nature of JavaScript
The inheritance model in JavaScript always raises many questions. It can be confusing, especially for programmers coming from class-based languages, like Java, C# or Python. In this article, we take a closer look at the prototypal nature of JavaScript.
How to create objects in JavaScript?
JavaScript supports many paradigms. One of them is object-oriented programming. In contrast to other known OOP languages, JavaScript doesn't have the concept of classes. Inheritance is done using prototypes.
Class-based languages | Prototype-based languages |
---|---|
Java, C#, C++, Python, Ruby | JavaScript |
How then are we supposed to create objects if there are no classes? Well, there is a couple of options.
To create a single object, you can use the object literal syntax. Just put a bunch of properties between curly braces and assign it to a variable.
var john = {
name: 'John',
lastName: 'Doe',
yearOfBirth: 1990
}
Now, you can access these properties, either with dot or bracket notation.
// Dot notation
john.name
// Bracket notation
john['name']
It works fine when you want a single object, but what if you need many similar objects? In that situation, use a function constructor.
So-called function constructors are just regular functions, but their responsibility is to create new objects. You can think about them as blueprints.
function Person(name, lastName, yearOfBirth) {
this.name = name;
this.lastName = lastName;
this.yearOfBirth = yearOfBirth;
}
To create an object with that constructor, use the
new
operator.
const john = new Person('John', 'Doe', 1990);
The new
operator creates an empty object, passes
it to the
constructor function as the value of this
and links the newly created object with the prototype
object of the Person constructor. More about that later.
In return, we get the object with properties defined inside the Person constructor, so the name, lastName, and yearOfBirth.
What is the prototype?
In JavaScript, every object has its prototype, but only functions have the prototype property.
I know this sentence sounds confusing, so let me break it down into two parts.
Functions have the prototype property. What exactly does it mean? As you may have heard, functions in JavaScript, are a special type of objects. It means they can have properties, like every other object. One of these properties is the prototype property, which by default, stores a reference to an empty object.
function Person(name, lastName, yearOfBirth) {
this.name = name;
this.lastName = lastName;
this.yearOfBirth = yearOfBirth;
}
console.log(Person.prototype); // empty object
We can fill this empty object with properties and methods that are inherited by the instances of the Person constructor.
function Person(name, lastName, yearOfBirth) {
this.name = name;
this.lastName = lastName;
this.yearOfBirth = yearOfBirth;
}
Person.prototype.calcAge = function() {
const year = new Date().getFullYear();
return year - this.yearOfBirth;
}
const john = new Person('John', 'Doe', 1990);
console.log(john.calcAge()); // 29
The john object inherits the calcAge
method
because it's an
instance of the Person constructor. We can create other objects using the same constructor, and all of them
inherit the calcAge
method.
const john = new Person('John', 'Doe', 1990);
const mike = new Person('Mike', 'Smith', 1995);
const jane = new Person('Jane', 'Wilson', 2000);
console.log(john.calcAge()); // 29
console.log(mike.calcAge()); // 24
console.log(jane.calcAge()); // 19
Let's take care of the second part of the sentence. Every object has its prototype. It means that in JavaScript, every possible object is an instance of some constructor, and inherits from the prototype object of this constructor.
Remember when the john object inherited the calcAge
method from the
Person.prototype
object? The same thing applies to every single object in JavaScript.
Take a look at arrays. You can create an array using the literal syntax (square brackets), but you can also use the Array constructor.
// Array literal syntax
const arr = [1, 2, 3];
// Array constructor
const arr2 = new Array(1, 2, 3);
Both lines create arrays with three elements. What does it mean? That arrays are objects, instances of the Array constructor.
It also means that arrays inherit from the Array.prototype
. I'm sure you know
the forEach
method used to iterate through the array. Guess what? This method comes from the
Array.prototype
.
[1, 2, 3].forEach(el => {
console.log(el);
});
Additionally, you can always check the prototype of an object
with Object.getPrototypeOf()
method.
const arr = [1, 2, 3];
console.log(Object.getPrototypeOf(arr));
Prototypes have their prototypes
If prototypes are objects, does it mean they also have their prototypes? And if so, what is the prototype of the prototype?
We know that prototypes are just simple objects. We also know that every single object in
JavaScript has
its prototype, from which it inherits properties and methods. All objects in JavaScript are descended
from Object
, which means they inherit from the Object.prototype
.
To prove this, create an empty object and check its prototype.
console.log(Object.getPrototypeOf({}));
The same thing applies to functions. Functions are a special
type of objects,
which means they are created with the Function
constructor and inherit from the Function.prototype
.
The prototype chain
Let's take a look at how the JavaScript interpreter finds the method in the chain of the prototypes.
function Person(name, lastName, yearOfBirth) {
this.name = name;
this.lastName = lastName;
this.yearOfBirth = yearOfBirth;
}
const john = new Person('John', 'Doe', 1990);
console.log(john.toString());
We have the john object that is an instance of the Person
constructor, which
means it inherits from the Person.prototype
object. We want to call the toString
method, which sits in the Object.prototype
. How is the interpreter able to find this method?
The JavaScript interpreter first checks if the toString
method exists in the
john object. It
doesn't, so the interpreter looks for this method in the Person.prototype
object. It can't
find it here, so it moves up to the next prototype in the chain, which is the
Object.prototype
. It finally finds the toString
there and calls this method
on the john object.
Why use prototypes?
Why would I even place a method in the prototype if I can just put it in the constructor function?
That's true, but there are benefits behind prototypes. Compare these two code samples.
function Person(name, lastName, yearOfBirth) {
this.name = name;
this.lastName = lastName;
this.yearOfBirth = yearOfBirth;
this.calcAge = function() {
const currentYear = new Date().getFullYear();
return currentYear - this.yearOfBirth;
}
}
const john = new Person('John', 'Doe', 1990);
console.log(john);
In this example, the calcAge
method is added
directly to the
instance of the Person constructor. Use the console.log()
method to see that the
calcAge
belongs to the john object.
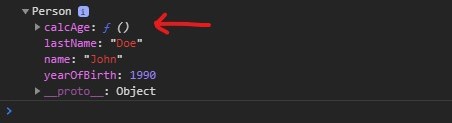
From the other hand, we have this code.
function Person(name, lastName, yearOfBirth) {
this.name = name;
this.lastName = lastName;
this.yearOfBirth = yearOfBirth;
}
Person.prototype.calcAge = function() {
const currentYear = new Date().getFullYear();
return currentYear - this.yearOfBirth;
}
const john = new Person('John', 'Doe', 1990);
console.log(john);
The calcAge
method sits in the prototype and is
inherited by the
instances of the Person constructor. The john object doesn't own the calcAge
method, but it can
access it through the prototype chain.
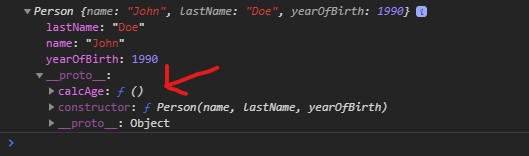
In most cases, using prototypes is more efficient and saves up memory because a method is declared only once for all objects created with a constructor, instead of being declared for each instance separately.
The __proto__ property
In the last example, you might have seen the strange-looking __proto__
property.
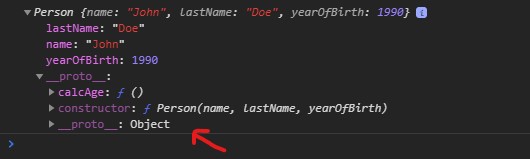
__proto__
propertyThe __proto__
property is a function that allows us to get and set the
prototype of an
object. In the case of the john object, the __proto__
returns the
Person.prototype
object.
Note that the use of the __proto__
property is not recommended anymore. To get
a prototype
of an object, use the Object.getPrototypeOf()
method.
The Object.create() method
In some cases, you may want to specify the prototype for your newly created object without
declaring any
constructor functions. The Object
has a special method for that.
const john = {
name: 'John',
lastName: 'Doe',
yearOfBirth: 1990,
calcAge: function() {
const currentYear = new Date().getFullYear();
return currentYear - this.yearOfBirth;
}
}
const mike = Object.create(john);
mike.name = 'Mike';
mike.lastName = 'Smith';
mike.yearOfBirth = 1995;
console.log(mike.calcAge());
The Object.create()
method allows us to specify
the prototype
for the newly created object. In this case, the mike object uses the john object as its prototype, which
allows Mike to use the calcAge
method.
This method is useful to implement so-called classical inheritance known from the class-based languages, but more on that in another article.
Classes in JavaScript
We determined earlier that JavaScript is a prototype-based language that doesn't have the concept of classes. However, the ES6 standard introduces a new class syntax to JavaScript.
class Person {
constructor(name, lastName, yearOfBirth) {
this.name = name;
this.lastName = lastName;
this.yearOfBirth = yearOfBirth;
}
calcAge() {
const currentYear = new Date().getFullYear();
return currentYear - this.yearOfBirth;
}
}
Now, you can use classes in JavaScript. However, they are just syntactical sugar, which means that behind the scenes, JavaScript is still based on prototypes. It's just a syntax that makes writing code more straightforward, but also hides the prototypal nature of JavaScript from new developers.
Summary
In this article, we've determined that JavaScript is a prototype-based language. Prototypes are simple objects used for inheritance. One object can inherit properties and methods from the other. Usually, using prototypes is more efficient and saves up memory because a method is declared only once, in a prototype. JavaScript doesn't have a true concept of classes known from the class-based languages, but we can use the class syntax.